Selecting A Rate
In order to ship with a carrier you first need to select a rate. To do this you can call our rates
endpoint to retrieve a list of rates and services. This endpoint is especially useful if you want to provide shipping quotes prior to an order being placed. A typical example of this would be displaying rates live for a customer to select from at checkout.
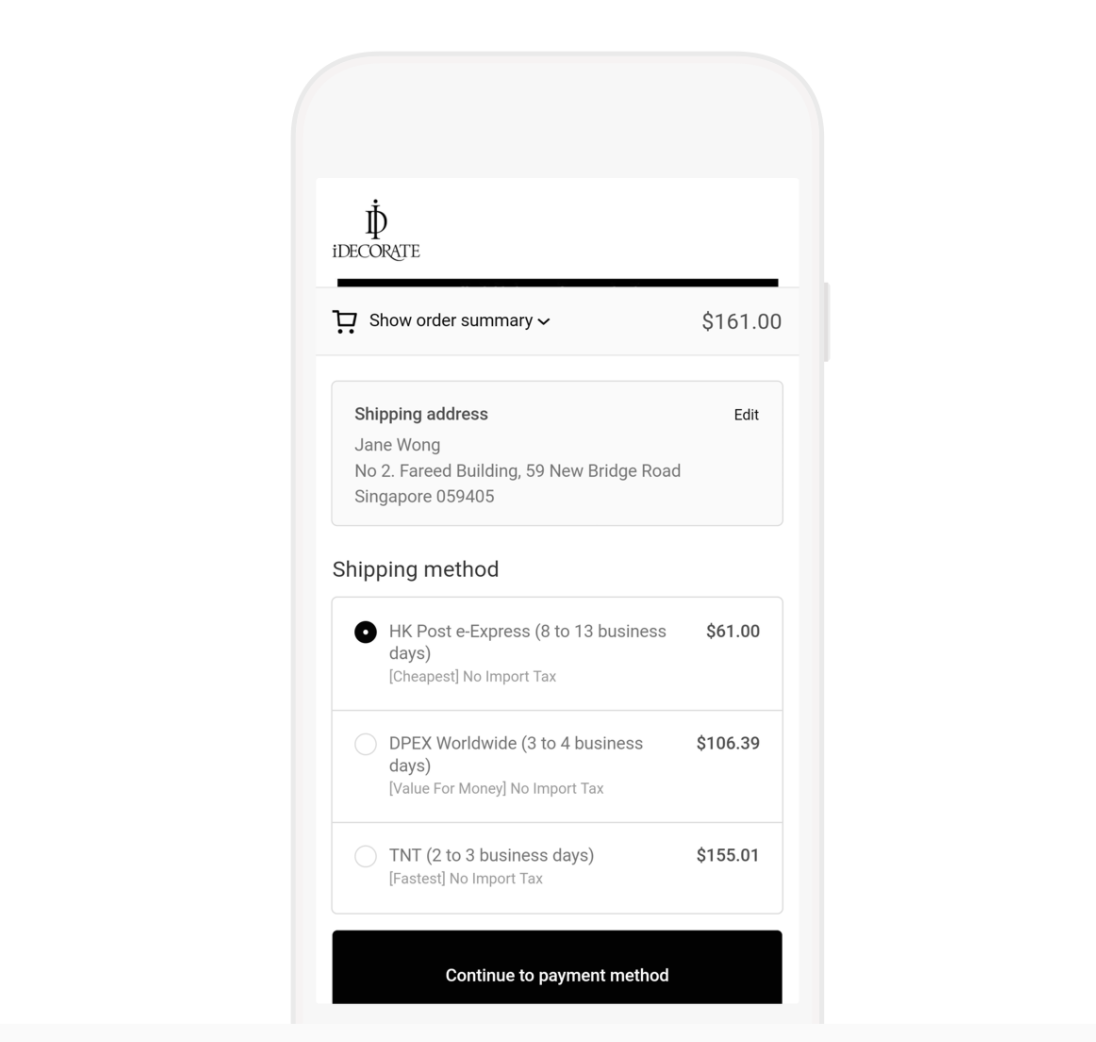
Live shipping rates in a shopping cart
1. Request a rate
In order to retrieve a list of rates, you need to first provide information on the product you are intending to ship. This includes the addresses and parcel information.
When requesting a rate, you need to include origin_postal_code
, destination_country_alpha2
& destination_postal_code
. You can also denote whether the duty is paid by the sender or receiver and if it is insured. As a default, it is set to the sender and no insurance included.
The object has a number of required attributes (including weight, dimensions and product category), which can be found in the API Reference here
{
"origin_postal_code": "WC2N",
"destination_country_alpha2": "US",
"destination_postal_code": "10030",
"taxes_duties_paid_by": "Sender",
"is_insured": false,
"items": [
{
"actual_weight": 1.2,
"height": 10,
"width": 15,
"length": 20,
"category": "mobiles",
"declared_currency": "SGD",
"declared_customs_value": 100
}
]
}
curl --include \
--request POST \
--header "Content-Type: application/json" \
--header "Authorization: Bearer <YOUR EASYSHIP API TOKEN>" \
--data-binary "{
\"origin_country_alpha2\": \"SG\",
\"origin_postal_code\": \"WC2N\",
\"destination_country_alpha2\": \"US\",
\"destination_postal_code\": \"10030\",
\"taxes_duties_paid_by\": \"Sender\",
\"is_insured\": false,
\"items\": [
{
\"actual_weight\": 1.2,
\"height\": 10,
\"width\": 15,
\"length\": 20,
\"category\": \"mobiles\",
\"declared_currency\": \"SGD\",
\"declared_customs_value\": 100
}
]
}" \
'https://api.easyship.com/rate/v1/rates'
require 'uri'
require 'net/http'
url = URI("https://api.easyship.com/rate/v1/rates")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTPrequire 'rubygems' if RUBY_VERSION < '1.9'
require 'rest_client'
values = '{
"origin_country_alpha2": "SG",
"origin_postal_code": "WC2N",
"destination_country_alpha2": "US",
"destination_postal_code": "10030",
"taxes_duties_paid_by": "Sender",
"is_insured": false,
"items": [
{
"actual_weight": 1.2,
"height": 10,
"width": 15,
"length": 20,
"category": "mobiles",
"declared_currency": "SGD",
"declared_customs_value": 100
}
]
}'
headers = {
:content_type => 'application/json',
:authorization => 'Bearer <YOUR EASYSHIP API TOKEN>'
}
response = RestClient.post 'https://api.easyship.com/rate/v1/rates', values, headers
puts response::Post.new(url)
request.body = "{\"origin_country_alpha2\":\"SG\",\"origin_postal_code\":\"WC2N\",\"destination_country_alpha2\":\"US\",\"destination_postal_code\":\"10030\",\"taxes_duties_paid_by\":\"sender\",\"is_insured\":\"false\",\"items\":{\"actual_weight\":1.2,\"height\":10,\"width\":15,\"length\":20,\"category\":\"mobiles\",\"declared_currency\":\"sgd\",\"declared_customs_value\":100}}"
response = http.request(request)
puts response.read_body
var request = require('request');
request({
method: 'POST',
url: 'https://api.easyship.com/rate/v1/rates',
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer <YOUR EASYSHIP API TOKEN>'
},
body: "{
\"origin_country_alpha2\": \"SG\",
\"origin_postal_code\": \"WC2N\",
\"destination_country_alpha2\": \"US\",
\"destination_postal_code\": \"10030\",
\"taxes_duties_paid_by\": \"Sender\",
\"is_insured\": false,
\"items\": [ {
\"actual_weight\": 1.2,
\"height\": 10,
\"width\": 15,
\"length\": 20,
\"category\":
\"mobiles\",
\"declared_currency\": \"SGD\",
\"declared_customs_value\": 100 } ]}"
}, function (error, response, body) {
console.log('Status:', response.statusCode);
console.log('Headers:', JSON.stringify(response.headers));
console.log('Response:', body);
});
from urllib2 import Request, urlopen
values = """
{
"origin_country_alpha2": "SG",
"origin_postal_code": "WC2N",
"destination_country_alpha2": "US",
"destination_postal_code": "10030",
"taxes_duties_paid_by": "Sender",
"is_insured": false,
"items": [
{
"actual_weight": 1.2,
"height": 10,
"width": 15,
"length": 20,
"category": "mobiles",
"declared_currency": "SGD",
"declared_customs_value": 100
}
]
}
"""
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer <YOUR EASYSHIP API TOKEN>'
}
request = Request('https://api.easyship.com/rate/v1/rates', data=values, headers=headers)
response_body = urlopen(request).read()
print response_body
<?php
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://api.easyship.com/rate/v1/rates");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);
curl_setopt($ch, CURLOPT_HEADER, FALSE);
curl_setopt($ch, CURLOPT_POST, TRUE);
curl_setopt($ch, CURLOPT_POSTFIELDS, "{
\"origin_country_alpha2\": \"SG\",
\"origin_postal_code\": \"WC2N\",
\"destination_country_alpha2\": \"US\",
\"destination_postal_code\": \"10030\",
\"taxes_duties_paid_by\": \"Sender\",
\"is_insured\": false,
\"items\": [
{
\"actual_weight\": 1.2,
\"height\": 10,
\"width\": 15,
\"length\": 20,
\"category\": \"mobiles\",
\"declared_currency\": \"SGD\",
\"declared_customs_value\": 100
}
]
}");
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
"Content-Type: application/json",
"Authorization: Bearer <YOUR EASYSHIP API TOKEN>"
));
$response = curl_exec($ch);
curl_close($ch);
var_dump($response);
The API will respond with a list of rates
{
"rates": [
{
"courier_id": "8ea55285-d53f-4b94-b788-17c4e5eefb5e",
"courier_name": "Singapore Post - ePack",
"min_delivery_time": 5,
"max_delivery_time": 7,
"value_for_money_rank": 2,
"delivery_time_rank": 7,
"shipment_charge": 30.38,
"fuel_surcharge": 0,
"remote_area_surcharge": null,
"shipment_charge_total": 30.38,
"warehouse_handling_fee": 0,
"insurance_fee": 0,
"ddp_handling_fee": 0,
"import_tax_charge": 0,
"import_duty_charge": 0,
"total_charge": 30.38,
"is_above_threshold": false,
"effective_incoterms": "DDU",
"estimated_import_tax": 0,
"estimated_import_duty": 0,
"courier_does_pickup": false,
"courier_dropoff_url": "https://www.easyship.com/drop-off/directlink/sg",
"tracking_rating": 2,
"payment_recipient": "Easyship",
"courier_remarks": null,
"currency": "SGD",
"box": {
"name": null,
"length": 20,
"width": 15,
"height": 10
}
},
{
"courier_id": "16691987-a149-441b-a777-727ffe5c3325",
"courier_name": "UPS Worldwide Saver - Battery",
"min_delivery_time": 2,
"max_delivery_time": 5,
"value_for_money_rank": 1,
"delivery_time_rank": 4,
"shipment_charge": 52.08,
"fuel_surcharge": 5.859,
"remote_area_surcharge": 0,
"shipment_charge_total": 57.939,
"warehouse_handling_fee": 0,
"insurance_fee": 0,
"ddp_handling_fee": 0,
"import_tax_charge": 0,
"import_duty_charge": 0,
"total_charge": 57.94,
"is_above_threshold": false,
"effective_incoterms": "DDU",
"estimated_import_tax": 0,
"estimated_import_duty": 0,
"courier_does_pickup": true,
"courier_dropoff_url": null,
"tracking_rating": 3,
"payment_recipient": "Easyship",
"courier_remarks": null,
"currency": "SGD",
"box": {
"name": null,
"length": 20,
"width": 15,
"height": 10
}
},
{
"courier_id": "200ff5db-6866-4fb9-851d-8943e8796b16",
"courier_name": "Singapore Post - Airmail",
"min_delivery_time": 6,
"max_delivery_time": 8,
"value_for_money_rank": 3,
"delivery_time_rank": 9,
"shipment_charge": 43.6,
"fuel_surcharge": 0,
"remote_area_surcharge": null,
"shipment_charge_total": 43.6,
"warehouse_handling_fee": 0,
"insurance_fee": 0,
"ddp_handling_fee": 0,
"import_tax_charge": 0,
"import_duty_charge": 0,
"total_charge": 43.6,
"is_above_threshold": false,
"effective_incoterms": "DDU",
"estimated_import_tax": 0,
"estimated_import_duty": 0,
"courier_does_pickup": false,
"courier_dropoff_url": "https://www.easyship.com/drop-off/directlink/sg",
"tracking_rating": 2,
"payment_recipient": "Easyship",
"courier_remarks": null,
"currency": "SGD",
"box": {
"name": null,
"length": 20,
"width": 15,
"height": 10
}
},
]
}
2. Select Your Preferred Rate
Once you have your rates you will also receive a number of related to the service level of the couriers. You will normally receive them ordered by value_for_money_rank
. Alongside this, you'll also receive the courier_id
.
When you have selected the service to be used you can store and reuse the courier_id": "638ac885-8105-422c-b2cc-4c57152772f7
, to create the shipment in the Easyship system.
Updated over 5 years ago
Now you have your selected rate, you can now go on to create the shipment in Easyship.